How to create custom method in predefined class C#?
Custom Method C#, Add Existing Method to Predefined Class, C#, Advanced C#

In C#, you can create a custom method in a predefined class using extension methods. Extension methods allow you to add new methods to existing classes without modifying the original class's source code. This can be useful when you want to add functionality to classes from third-party libraries or system classes.
To create a custom method in a predefined class using an extension method, follow these steps:
-
Define a static class to contain your extension method. The class should be static and non-generic.
-
Define a static method within the static class, and use the
this
keyword with the first parameter to indicate which class you are extending. Thethis
parameter represents the instance of the class that the extension method will be applied to.
Here's a step-by-step guide with an example:
Suppose we want to create a custom method to calculate the square of an integer in the int
data type's predefined class.
- Create a new static class to hold the extension method:
public static class IntExtensions
{
// Custom extension method to calculate the square of an integer
public static int Square(this int number)
{
return number * number;
}
}
Now you can use the Square()
method on any integer variable as if it were a built-in method:
using System;
class Program
{
static void Main()
{
int myNumber = 5;
// Using the custom Square() method on the integer variable
int squaredValue = myNumber.Square();
Console.WriteLine($"Square of {myNumber} is: {squaredValue}");
}
}
Output:
Square of 5 is: 25
In the example above, we created a custom extension method Square()
for the int
data type. The method is defined in the IntExtensions
class, and it can now be called on any int
variable, just like any other built-in method.
Remember that extension methods must be defined in a static class and should be placed in a static namespace to be accessible throughout your application. Also, be mindful of naming conflicts and choose meaningful and unique names for your custom methods.
What's Your Reaction?
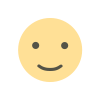
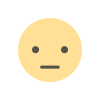
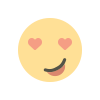
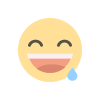
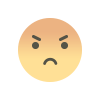
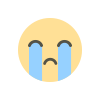
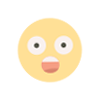